实现功能
根据B站发布的OneNET的HTTP接入方式,将LU9061所测得的温度数据发送到云端。
代码
自己写的部分已上下注释标出
`#include <unistd.h>
#include <stdlib.h>
#include <stdio.h>
#include <sys/socket.h>
#include <lwip/api.h>
#include <lwip/arch.h>
#include <lwip/opt.h>
#include <lwip/inet.h>
#include <lwip/errno.h>
#include <netdb.h>
#include "bflb_adc.h"
#include "shell.h"
#include "utils_getopt.h"
#include "bflb_mtimer.h"
#include "board.h"
#include "bflb_uart.h"
#include "log.h"
#define HOST_NAME "183.230.40.33"
#define ONENET_HTTP_POST_MAX_LEN 1024
#define ONENET_HTTP_POST_CONTENT_MAX_LEN 1024
#define DEV_ID "1103699461" //设备ID 更换为自己的设备id后再编译
#define API_KEY "bkhq6YaR=9Csyo98bliDGmBGXQE=" //API-KEY 更换为自己的API-KEY后再编译
/*****************定义部分*********************/
#define DBG_TAG "MAIN"
#define u8 unsigned char
#define u16 unsigned int
#define USART_REC_LEN 200
struct bflb_device_s *uartx;
static uint8_t uart_txbuf[128] = { 0 };
u16 USART_RX_LEN = 0;
u16 USART_RX_BUF[USART_REC_LEN];
u16 USART_RX_STA = 0;
u16 USART_STA = 0;
/***************************************************/
// clang-format off
// static const uint8_t get_buf[] = "GET / HTTP/1.1 \r\nHost: www.gov.cn\r\n\r\n";
uint32_t recv_buf[4 * 1024] = { 0 };
// clang-format on
shell_sig_func_ptr abort_exec;
uint64_t total_cnt = 0;
int sock_client = -1;
/*************************UART配置**************************/
void uart_isr(int irq, void *arg)
{
u16 Res;
uint32_t intstatus = bflb_uart_get_intstatus(uartx);
if (intstatus & UART_INTSTS_RX_FIFO) {
// printf("rx fifo\r\n");
while (bflb_uart_rxavailable(uartx)) {
if((USART_RX_STA & 0x8000)==0)
{
// printf("fifo test1\r\n");
Res = bflb_uart_getchar(uartx);
USART_RX_BUF[USART_RX_LEN]=Res;
USART_RX_LEN++;
// }
// if(USART_RX_STA==0)//½ÓÊÕδÍê³É
// {
if(Res==0Xfe)
{
USART_STA=1;
USART_RX_LEN=0;
// printf("fifo test2\r\n");
}
if(USART_STA)
{
USART_RX_BUF[USART_RX_LEN]=Res;
USART_RX_LEN++;
if(USART_RX_LEN>8)
{
USART_STA=0;
USART_RX_STA |= 0x8000;
// printf("fifo test4\r\n");
}
// printf("fifo test3\r\n");
}
}
// printf("0x%02x", bflb_uart_getchar(uartx));
// printf("0x%02x\r\n",Res);
// printf("fifo test\r\n");
}
// bflb_uart_rxint_mask(uartx, true);
bflb_uart_feature_control(uartx, UART_CMD_SET_RTS_VALUE, 1);
}
if (intstatus & UART_INTSTS_RTO) {
// printf("rto\r\n");
while (bflb_uart_rxavailable(uartx)) {
if((USART_RX_STA & 0x8000)==0)
{
// printf("fifo test1\r\n");
Res = bflb_uart_getchar(uartx);
USART_RX_BUF[USART_RX_LEN]=Res;
USART_RX_LEN++;
// }
// if(USART_RX_STA==0)//½ÓÊÕδÍê³É
// {
if(Res==0Xfe)
{
USART_STA=1;
USART_RX_LEN=0;
// printf("fifo test2\r\n");
}
if(USART_STA)
{
USART_RX_BUF[USART_RX_LEN]=Res;
USART_RX_LEN++;
if(USART_RX_LEN>8)
{
USART_STA=0;
USART_RX_STA |= 0x8000;
// printf("fifo test4\r\n");
}
// printf("fifo test3\r\n");
}
}
// printf("rto111\r\n");
}
bflb_uart_int_clear(uartx, UART_INTCLR_RTO);
}
if (intstatus & UART_INTSTS_TX_FIFO) {
// printf("tx fifo\r\n");
for (uint8_t i = 0; i < 27; i++) {
// bflb_uart_putchar(uartx, uart_txbuf[i]);
}
bflb_uart_txint_mask(uartx, true);
}
}
/*******************************************************************/
static void test_close(int sig)
{
if (sock_client) {
closesocket(sock_client);
}
abort_exec(sig);
if (total_cnt > 0) {
printf("Total send data=%lld\r\n", total_cnt);
}
}
#define PING_USAGE \
"wifi_http_test [hostname] [port]\r\n" \
"\t hostname: hostname or dest server ip\r\n" \
"\t port: dest server listen port, defualt port:80\r\n"
static void wifi_test_http_client_init(int argc, char **argv)
{
/*******在此处定义了WENDU_H 是传感器测得温度的整数部分********/
u8 WENDU_H , WENDU_L;
board_init();
board_uartx_gpio_init();
uartx = bflb_device_get_by_name(DEFAULT_TEST_UART);
struct bflb_uart_config_s cfg;
cfg.baudrate = 9600;
cfg.data_bits = UART_DATA_BITS_8;
cfg.stop_bits = UART_STOP_BITS_1;
cfg.parity = UART_PARITY_NONE;
cfg.flow_ctrl = 0;
cfg.tx_fifo_threshold = 8;
cfg.rx_fifo_threshold = 2;
bflb_uart_init(uartx, &cfg);
bflb_uart_txint_mask(uartx, false);
bflb_uart_rxint_mask(uartx, false);
bflb_irq_attach(uartx->irq_num, uart_isr, NULL);
bflb_irq_enable(uartx->irq_num);
bflb_uart_putchar(uartx, 0XFA);
bflb_uart_putchar(uartx, 0XC5);
bflb_uart_putchar(uartx, 0XBF);
bflb_mtimer_delay_ms(50);
/******************WENDU_L为小数部分*********************/
abort_exec = shell_signal(SHELL_SIGINT, test_close);
printf("Http client task start ...\r\n");
char *host_name;
char *addr;
char *port;
struct sockaddr_in remote_addr;
// if (argc < 2) {
// printf("%s", PING_USAGE);
// return;
// }
if (argc > 1) {
/* get address (argv[1] if present) */
host_name = argv[1];
#ifdef LWIP_DNS
ip4_addr_t dns_ip;
netconn_gethostbyname(host_name, &dns_ip);
addr = ip_ntoa(&dns_ip);
#endif
}
else {
addr = HOST_NAME;
}
/* get port number (argv[2] if present) */
if (argc > 2) {
port = argv[2];
} else {
port = "80";
}
while (1) {
if ((sock_client = socket(AF_INET, SOCK_STREAM, 0)) < 0) {
printf("Http Client create socket error\r\n");
return;
}
//准备数据
/**********************此处输出测得的数据******************/
for (uint8_t i = 0; i < 128; i++) {
uart_txbuf = i;
}
if(USART_RX_STA&0X8000)//
{
// printf("wendu2 : ");
WENDU_H = USART_RX_BUF[2];
WENDU_L = USART_RX_BUF[3]/10;
WENDU_H = WENDU_H & 0X00FF;
// OLED_ShowNum(80,6,WENDU_H,2,1);//
// OLED_ShowNum(104,6,WENDU_L,1,1);//
printf("%d.%d\r\n", WENDU_H,WENDU_L);
// printf("0x%02x\r\n", bflb_uart_getchar(uartx));
USART_RX_STA = 0;//
}
bflb_mtimer_delay_ms(200);
/*************************************************************/
remote_addr.sin_family = AF_INET;
remote_addr.sin_port = htons(atoi(port));
remote_addr.sin_addr.s_addr = inet_addr(addr);
memset(&(remote_addr.sin_zero), 0, sizeof(remote_addr.sin_zero));
printf("Host:%s, Server ip Address : %s:%s\r\n", HOST_NAME, addr, port);
if (connect(sock_client, (struct sockaddr *)&remote_addr, sizeof(struct sockaddr)) != 0) {
printf("Http client connect server falied!\r\n");
closesocket(sock_client);
return;
}
printf("Http client connect server success!\r\n");
printf("Press CTRL-C to exit.\r\n");
memset(recv_buf, 0, sizeof(recv_buf));
total_cnt = 0;
//创建post数据
char post_buf[ONENET_HTTP_POST_MAX_LEN];
char post_content[ONENET_HTTP_POST_CONTENT_MAX_LEN];
char post_content_len[4];
memset(post_content, 0, sizeof(post_content));
memset(post_buf, 0, sizeof(post_buf));
sprintf(post_content,"{\"datastreams\":["
"{\"id\":\"WENDU_H\",\"datapoints\":[{\"value\":%d}]},"
"]}",WENDU_H);
sprintf(post_content_len,"%d",strlen(post_content));
strcat(post_buf, "POST /devices/");
strcat(post_buf, DEV_ID);
strcat(post_buf, "/datapoints HTTP/1.1\r\n");
strcat(post_buf, "api-key:");
strcat(post_buf, API_KEY);
strcat(post_buf, "\r\n");
strcat(post_buf, "Host:api.heclouds.com\r\n");
strcat(post_buf, "Content-Length:");
strcat(post_buf, post_content_len);
strcat(post_buf, "\r\n\r\n");
strcat(post_buf, post_content);
strcat(post_buf, "\r\n\r\n");
printf("%s\r\n", post_buf);
write(sock_client, post_buf, sizeof(post_buf));
while (1) {
total_cnt = recv(sock_client, (uint8_t *)recv_buf, sizeof(recv_buf), 0);
if (total_cnt <= 0)
break;
printf("%s\r\n", (uint8_t *)recv_buf);
vTaskDelay(5000);
}
closesocket(sock_client);
// return;
}
}
#ifdef CONFIG_SHELL
#include <shell.h>
int cmd_wifi_http_client(int argc, char **argv)
{
wifi_test_http_client_init(argc, argv);
return 0;
}
SHELL_CMD_EXPORT_ALIAS(cmd_wifi_http_client, onenet_post, wifi http client test);
#endif`
- 出现的问题
连接wifi后发送数据,Xshell显示中断未注册
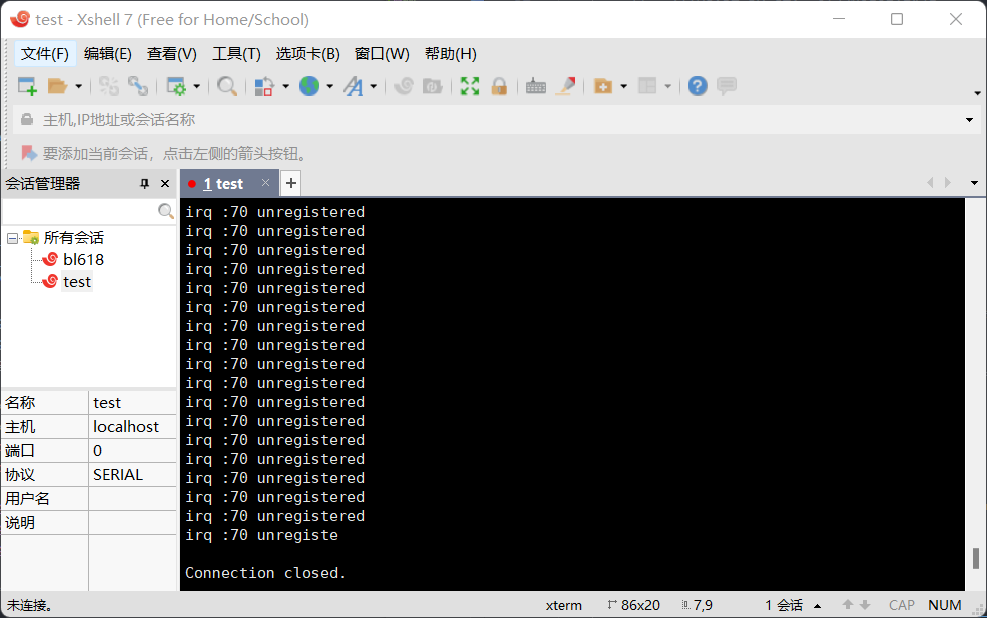