本篇文章由 VeriMake 旧版论坛中备份出的原帖的 Markdown 源码生成
原帖标题为:Using a Touch Sensor to Play Chrome's Dino game
原帖网址为:https://verimake.com/topics/83 (旧版论坛网址,已失效)
原帖作者为:Maggie(旧版论坛 id = 83,注册于 2020-04-26 09:34:15)
原帖由作者初次发表于 2020-05-03 16:39:32,最后编辑于 2020-05-03 16:39:32(编辑时间可能不准确)
截至 2021-12-18 14:27:30 备份数据库时,原帖已获得 1463 次浏览、2 个点赞、0 条回复
Introduction
This project was inspired by Automated Dino Game Using LDR on Arduino Project Hub. It's like a cheating program that automatically plays the game for you.
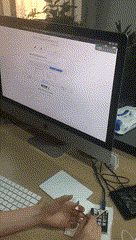
In this project, you can control the jumping of the little dinosaur in the game with your touch on the sensor (you still have to play the game yourself).
Hardware Preparation
✔ Arduino ✔ Base Shield ✔Grove-Touch_Sensor
- Connect Grove-Touch_Sensor to port D2 of Grove-Base Shield.
- Plug Grove - Base Shield into Arduino.
- Connect Arduino to PC via a USB cable
Reference: http://wiki.seeedstudio.com/Grove-Touch_Sensor/
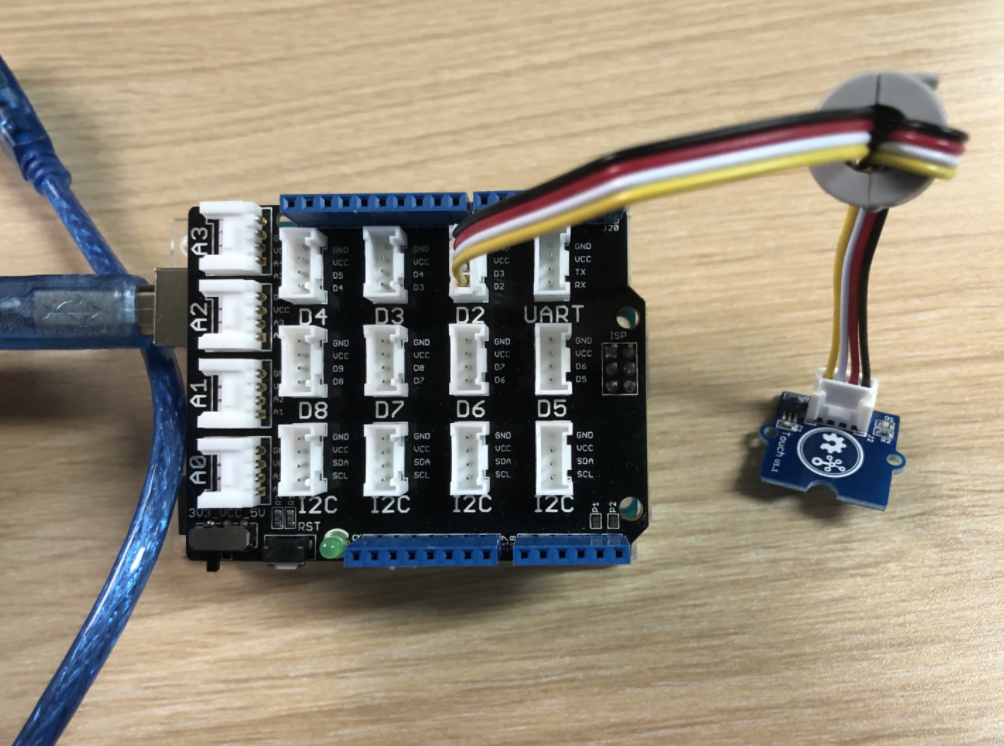
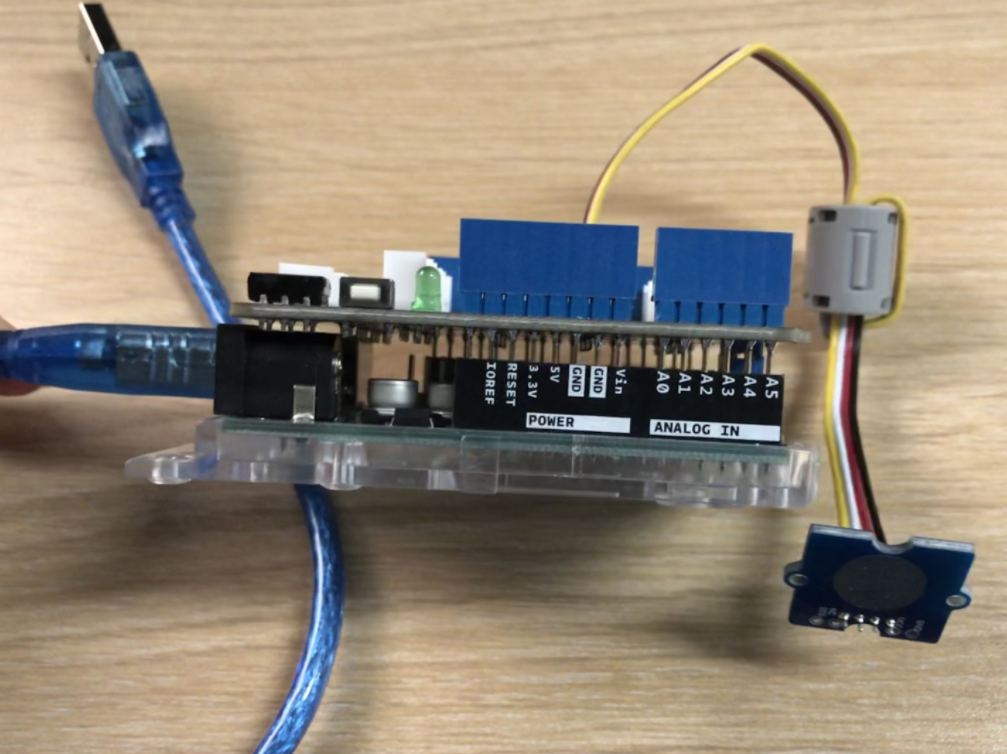
Codes on Arduino
// Arduino Dino Game ! :)
const int TouchPin = 2; // set the port where you connected your touch sensor
int sensorValue = 0; // variable to store the value coming from your touch sensor
int thresholdValue = 45; //this one depend on your trial and error method.
void setup() {
Serial.begin(9600);
}
void loop() {
// read the value from the sensor:
int sensorValue = digitalRead(TouchPin);
Serial.println(sensorValue); //uncomment this while first use and take your thresholdValue and set.
if(sensorValue==1){
delay(40); //delay 50ms
}
}
Codes on Python
import subprocess
import time
import pyautogui
import serial #See Error Prone Codes[1] below if you could not import this module
from serial import SerialException
#uncomment it if you don't have chrome in your computer. If you have chrome, open the web page #'chrome://dino' and directly run the code
#subprocess.call([r'/Applications/safari.app', '_blank', 'https://chromedino.com/'])
time.sleep(6) #give a short time to open and setup all.
print("All sett :)")
#Update with your arduino [port]
ser = serial.Serial('/dev/cu.usbmodem14201')
#set Serial Baud Rate at the baud rate of your sensor (in my case it's 9600).
ser.baudrate = '9600'
# Baud rate roughly means the speed that data is transmitted,
# and it is a derived value based on the number of symbols transmitted per second.
while True: # looping.
try:
h1=ser.readline() #reading serial data.
#decode and make it an int value. See Error prone codes[2] below
ss = ord(h1.decode().strip())
#print(ss) uncomment this line if you want to check the value of ss
if ss == 49: # true while obstacle.
print("Oh :< Jump!! ")
#print(ss) uncomment this line if you want to check the value of ss
pyautogui.press('space') #Auto press [space] key
except serialException:
print(error) # Maybe don't do this, or mess around with the interval
continue
Error Prone Codes
1.If you don't have the module "serial ", type this in your terminal instead of "pip install serial"
pip install pyserial
2.If we directly print(h1) without any decoding, the output will be like below
b'0\r\n' #this is when the touch sensor doesn't sense your fingers
b'0\r\n'
b'1\r\n' #this is when the touch sensor senses your fingers
b'1\r\n'
# The return value is the corresponding decimal integer
Python prints a TXT file with b' \r\n', so if we only want the return value of 0 or 1, we need to decode it with .decode().strip() after the variable
#add .decode().strip() after the variable you want to decode, and then t ransform the datatype from str to int
ss = ord(h1.decode().strip())
print(ss)
----------------------------------------------------------------------------------------------
48 #this is when the touch sensor doesn't sense your fingers
48
49 #this is when the touch sensor senses your fingers
49
The return value is no longer 0 or 1, but 48 and 49, this is because we used the method ord(), which returns an integer representing Unicode code point for the given Unicode character.
the corresponding **Unicode character for 0 is 48 **and the corresponding Unicode character for 1 is 49 (check ASCII table for further information)
Bibliography
- https://segmentfault.com/q/1010000013505625
- https://www.setra.com/blog/what-is-baud-rate-and-what-cable-length-is-required-1